|
|
|
Loops
Loops are used to repeat a block of code. You should
understand the concept of C++'s true and false, because it
will be necessary when working with loops.There are three
types of loops: for, while, (and) do while. Each of them has
their specific uses. They are all outlined below.
FOR - for loops are the most useful type. The layout is
for(variable initialization, conditional expression,
modification of variable) The variable initialization allows
you to either declare a variable and give it a value or give a
value to an already declared variable. Second, the conditional
expression tells the program that while the conditional
expression is true the loop should continue to repeat itself.
The variable modification section is the easiest way for a for
loop to handle changing of the variable. It is possible to do
things like x++, x=x+10, or even x=random(5);, and if you
really wanted to, you could call other functions that do
nothing to the variable. That would be something ridiculous
probably.
Example:
#include
//We only need one header file
int main() //We always need this
{ //The loop goes while x<100, and x increases by one every
loop
for(int x=0;x<100;x++) //Keep in mind that the loop
condition checks
{ //the conditional statement before it loops again.
//consequently, when x equals 100 the loop breaks
cout<
}
return 0;
}
This program is a very simple example of a for loop. x is set
to zero, while x is less than 100 it calls cout<
Example:
#include
//We only need this header file
int main() //Of course...
{
int x=0; //Don't forget to declare variables
while(x<100) //While x is less than 100 do
{
cout<
x++; //Adds 1 to x every time it repeats, in for loops the
//loop structure allows this to be done in the structure
}
return 0;
}
This was another simple example, but it is longer than the
above FOR loop. The easiest way to think of the loop is to
think the code executes, when it reaches the brace at the end
it goes all the way back up to while, which checks the boolean
expression.
DO WHILE - DO WHILE loops are useful for only things that want
to loop at least once. The structure is DO {THIS} WHILE
(TRUE);
Example:
#include
int main()
{
int x;
x=0;
do
{
cout<<"Hello world!";
}while(x!=0); //Loop while x is not zero, ut first execute the
//code in the section. (It outputs "Hello..." once
return 0;
}
Keep in mind that you must include a trailing semi-colon after
while in the above example. Notice that this loop will also
execute once, because it automatically executes before
checking the truth statement.
-kolij-
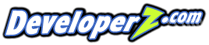
|
|